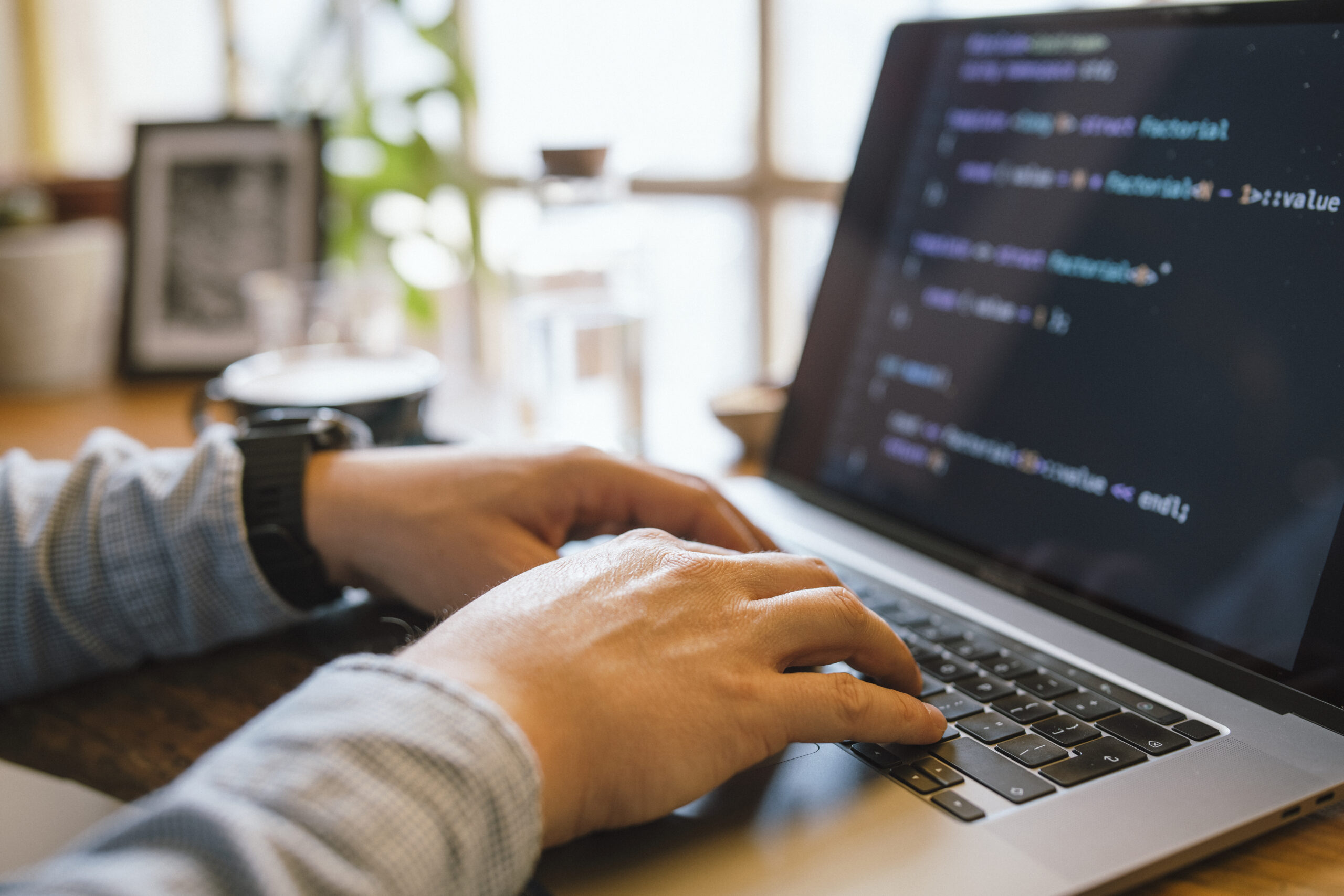
Debugging is Among the most essential — nevertheless generally missed — competencies in a developer’s toolkit. It isn't nearly fixing broken code; it’s about knowing how and why things go wrong, and Studying to Feel methodically to solve difficulties proficiently. Irrespective of whether you are a starter or simply a seasoned developer, sharpening your debugging competencies can help you save several hours of aggravation and significantly enhance your productivity. Here are several procedures to assist developers amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Equipment
One of many fastest techniques developers can elevate their debugging skills is by mastering the applications they use on a daily basis. Even though producing code is one particular Portion of improvement, realizing how you can connect with it proficiently during execution is Similarly critical. Contemporary development environments appear Outfitted with powerful debugging abilities — but several developers only scratch the area of what these instruments can do.
Just take, as an example, an Integrated Progress Setting (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment assist you to established breakpoints, inspect the value of variables at runtime, step as a result of code line by line, and in some cases modify code around the fly. When used appropriately, they let you notice precisely how your code behaves during execution, and that is priceless for tracking down elusive bugs.
Browser developer tools, for instance Chrome DevTools, are indispensable for front-conclusion developers. They help you inspect the DOM, keep track of community requests, check out real-time functionality metrics, and debug JavaScript within the browser. Mastering the console, sources, and community tabs can change disheartening UI problems into workable responsibilities.
For backend or method-stage builders, equipment like GDB (GNU Debugger), Valgrind, or LLDB present deep control above jogging processes and memory management. Discovering these resources could have a steeper Mastering curve but pays off when debugging performance concerns, memory leaks, or segmentation faults.
Outside of your IDE or debugger, come to be relaxed with Model Command methods like Git to comprehend code historical past, come across the precise moment bugs had been launched, and isolate problematic alterations.
Finally, mastering your applications means heading outside of default configurations and shortcuts — it’s about producing an personal expertise in your enhancement environment to ensure when difficulties occur, you’re not missing in the dead of night. The greater you already know your instruments, the greater time you are able to invest solving the actual problem rather than fumbling via the method.
Reproduce the challenge
Among the most important — and infrequently forgotten — techniques in powerful debugging is reproducing the condition. Right before leaping to the code or generating guesses, developers need to produce a constant environment or state of affairs wherever the bug reliably appears. With out reproducibility, fixing a bug gets to be a game of prospect, generally resulting in wasted time and fragile code variations.
The initial step in reproducing a difficulty is collecting as much context as possible. Talk to issues like: What actions triggered The problem? Which natural environment was it in — advancement, staging, or production? Are there any logs, screenshots, or mistake messages? The more depth you've, the a lot easier it results in being to isolate the exact conditions underneath which the bug occurs.
When you finally’ve collected plenty of facts, make an effort to recreate the problem in your neighborhood environment. This might necessarily mean inputting precisely the same info, simulating identical user interactions, or mimicking process states. If the issue appears intermittently, take into consideration composing automated assessments that replicate the sting instances or condition transitions associated. These tests not simply help expose the situation but additionally avert regressions Down the road.
At times, The problem may be surroundings-specific — it might come about only on sure operating programs, browsers, or underneath particular configurations. Making use of applications like virtual machines, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating these types of bugs.
Reproducing the issue isn’t only a move — it’s a state of mind. It needs endurance, observation, and also a methodical solution. But once you can regularly recreate the bug, you are already halfway to fixing it. Having a reproducible scenario, You can utilize your debugging equipment far more successfully, check prospective fixes securely, and talk a lot more Obviously along with your crew or people. It turns an summary grievance into a concrete problem — and that’s exactly where developers prosper.
Study and Comprehend the Error Messages
Mistake messages in many cases are the most worthy clues a developer has when a thing goes Erroneous. In lieu of looking at them as discouraging interruptions, builders must discover to treat mistake messages as direct communications from your technique. They usually tell you what precisely took place, the place it occurred, and sometimes even why it happened — if you know the way to interpret them.
Start off by reading through the message thoroughly and in complete. Lots of builders, particularly when below time tension, glance at the very first line and straight away start off creating assumptions. But further from the error stack or logs may perhaps lie the real root trigger. Don’t just duplicate and paste error messages into search engines — examine and fully grasp them very first.
Crack the error down into pieces. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Will it point to a certain file and line quantity? What module or purpose triggered it? These inquiries can manual your investigation and place you toward the liable code.
It’s also useful to be aware of the terminology from the programming language or framework you’re working with. Error messages in languages like Python, JavaScript, or Java typically abide by predictable styles, and learning to recognize these can greatly quicken your debugging approach.
Some errors are vague or generic, As well as in Those people situations, it’s very important to examine the context through which the mistake happened. Verify relevant log entries, enter values, and recent alterations during the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger problems and provide hints about likely bugs.
In the end, error messages are certainly not your enemies—they’re your guides. Discovering to interpret them properly turns chaos into clarity, aiding you pinpoint troubles speedier, cut down debugging time, and become a far more successful and self-confident developer.
Use Logging Correctly
Logging is One of the more powerful tools inside a developer’s debugging toolkit. When employed proficiently, it offers authentic-time insights into how an software behaves, serving to you fully grasp what’s occurring beneath the hood while not having to pause execution or action through the code line by line.
A good logging strategy starts off with recognizing what to log and at what amount. Popular logging concentrations involve DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for thorough diagnostic details in the course of advancement, Information for general activities (like effective start-ups), Alert for probable troubles that don’t break the application, Mistake for true troubles, and Deadly when the system can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant info. An excessive amount of logging can obscure important messages and slow down your process. Target crucial occasions, point out alterations, input/output values, and important determination points inside your code.
Structure your log messages Obviously and consistently. Include things like context, including timestamps, ask for IDs, and function names, so it’s easier to trace difficulties in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to track how variables evolve, what ailments are satisfied, and what branches of logic are executed—all without the need of halting This system. They’re Specifically valuable in creation environments where stepping by way of code isn’t possible.
Moreover, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the long run, wise logging is about harmony and clarity. With a effectively-assumed-out logging strategy, you could reduce the time it requires to spot concerns, get deeper visibility into your programs, and Enhance the In general maintainability and reliability of the code.
Assume Similar to a Detective
Debugging is not simply a technological task—it's a kind of investigation. To proficiently identify and repair bugs, developers have to solution the method just like a detective fixing a secret. This mentality assists break down sophisticated troubles into workable sections and adhere to clues logically to uncover the basis lead to.
Start out by accumulating proof. Think about the indications of the problem: error messages, incorrect output, or performance difficulties. The same as a detective surveys against the law scene, accumulate just as much suitable facts as you could without the need of leaping to conclusions. Use logs, exam instances, and user reports to piece together a transparent photograph of what’s occurring.
Following, variety hypotheses. Check with you: What may be triggering this habits? Have any improvements just lately been manufactured on the codebase? Has this situation transpired just before below very similar situation? The intention will be to slim down opportunities and discover likely culprits.
Then, examination your theories systematically. Try and recreate the trouble in a very managed surroundings. In the event you suspect a selected functionality or part, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, check with your code queries and Allow the results guide you closer to the reality.
Pay out shut consideration to tiny details. Bugs generally conceal inside the the very least predicted locations—similar to a lacking semicolon, an off-by-one mistake, or perhaps a race affliction. Be comprehensive and client, resisting the urge to patch The problem with out thoroughly knowing it. Non permanent fixes might cover the real dilemma, just for it to resurface later.
And lastly, hold notes on what you experimented with and acquired. Just as detectives log their investigations, documenting your debugging course of action can save time for foreseeable future challenges and assist Some others understand your reasoning.
By thinking like a detective, developers can sharpen their analytical techniques, approach troubles methodically, and come to be more effective at uncovering hidden troubles in advanced systems.
Compose Checks
Writing exams is one of the best tips on how to improve your debugging expertise and Total progress performance. Checks not merely support capture bugs early but will also function a security Web that offers you confidence when creating adjustments to the codebase. A properly-analyzed software is much easier to debug mainly because it allows you to pinpoint exactly exactly where and when an issue occurs.
Start with unit tests, which focus on personal functions or modules. These little, isolated tests can rapidly reveal whether or not a specific bit of logic is Doing the job as envisioned. Every time a take a look at fails, you immediately know where by to glimpse, noticeably cutting down enough time put in debugging. Unit tests are Primarily practical for catching regression bugs—difficulties that reappear soon after Formerly currently being mounted.
Subsequent, combine integration assessments and stop-to-finish checks into your workflow. These enable be certain that different parts of your software do the job collectively easily. They’re particularly handy for catching bugs that take place in complicated units with a number of components or products and services interacting. If anything breaks, your tests can inform you which Component of the pipeline failed and less than what situations.
Writing assessments also forces you to definitely Consider critically about your code. To check a feature adequately, you will need to be familiar with its inputs, envisioned outputs, and edge circumstances. This volume of knowing The natural way qualified prospects to raised code structure and less bugs.
When debugging an issue, composing a failing exam that reproduces the bug could be a robust first step. When the test fails persistently, you could give attention to correcting the bug and watch your examination go when The difficulty is settled. This technique makes certain that exactly the same bug doesn’t return Down the road.
In brief, producing checks turns debugging from a aggravating guessing match right into a structured and predictable process—aiding you capture additional bugs, faster and even more reliably.
Just take Breaks
When debugging a tricky concern, it’s effortless to be immersed in the condition—staring at your screen for hours, making an attempt Resolution immediately after Alternative. But Probably the most underrated debugging resources is just stepping away. Having breaks allows you reset your intellect, reduce aggravation, and often see the issue from a new perspective.
When you're too close to the code for too lengthy, cognitive fatigue sets in. You could possibly start off overlooking clear problems or misreading code that you just wrote just hrs previously. In this particular condition, your brain gets to be much less efficient at problem-resolving. A brief wander, a espresso split, and even switching to a special job for ten–quarter-hour can refresh your emphasis. A lot of developers report finding the foundation of a challenge once they've taken time for you to disconnect, allowing their subconscious function in the history.
Breaks also support avoid burnout, Particularly during for a longer period debugging periods. Sitting before a display, mentally stuck, is don't just unproductive but in addition draining. Stepping away enables you to return with renewed Electrical power and also a clearer attitude. You might instantly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
For those who’re caught, a good guideline would be to established a timer—debug actively for 45–60 minutes, then take a five–10 moment break. Use that point to move all over, stretch, or do a thing unrelated to code. It may sense counterintuitive, Particularly underneath tight deadlines, but it surely in fact leads to speedier and more effective debugging Eventually.
In short, using breaks is not really a sign of weak point—it’s a sensible technique. It offers your Mind Area to breathe, enhances your standpoint, and assists you steer clear of the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Each bug you come across is a lot more than simply a temporary setback—It really is a chance to mature being a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural situation, every one can instruct you some thing useful when you go to the trouble to reflect and analyze what went Improper.
Start off by inquiring on your own a handful of crucial inquiries when the bug is fixed: What prompted it? Why did it go unnoticed? Could it have been caught earlier with better methods like unit testing, code critiques, or logging? The answers frequently reveal blind spots in your workflow or comprehending and assist you to Develop more robust coding practices transferring forward.
Documenting bugs can be a superb behavior. Maintain a developer journal or preserve a log where you note down bugs you’ve encountered, the way you solved them, and That which you figured out. After a while, you’ll start to see patterns—recurring issues or popular issues—that you can proactively avoid.
In workforce environments, sharing That which you've uncovered from a bug with your friends might be Specifically potent. Whether it’s via a Slack concept, a short produce-up, or a quick knowledge-sharing session, encouraging Other folks stay away from the very same problem boosts workforce effectiveness and cultivates a stronger Mastering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical areas of your development journey. In spite of everything, a number of the most effective developers are usually not those who create great code, but people who check here consistently find out from their issues.
Ultimately, Each individual bug you resolve provides a brand new layer to the skill set. So upcoming time you squash a bug, take a second to replicate—you’ll come away a smarter, additional capable developer as a consequence of it.
Conclusion
Strengthening your debugging competencies will take time, exercise, and patience — nevertheless the payoff is large. It will make you a more productive, self-assured, and able developer. The next time you are knee-deep in a very mysterious bug, remember: debugging isn’t a chore — it’s a chance to become superior at what you do.